Coding a trading bot using MQL4 and MQL5 is a powerful way to automate your trading strategy on the MetaTrader platforms. MQL4 is used for programming bots on MetaTrader 4 (MT4), while MQL5 is designed for MetaTrader 5 (MT5). Both languages offer robust features for creating custom trading algorithms, including built-in functions for technical analysis, order management, and risk controls. When coding a trading bot, it’s essential to understand the core structure of the platform, including events like OnTick, which is triggered on every new market price update, and OnInit, which initializes the bot’s settings. Once you’ve written your code, you can backtest it using the strategy tester in MetaTrader, which simulates trading based on historical data, helping you fine-tune your bot before deploying it in live markets.
For those using MetaTrader 4, platforms like Exness MT4 provide access to a stable and reliable environment for deploying your trading bots. MT4 offers various features for managing trades, such as automated execution, custom indicators, and real-time market data, which are essential when running a trading algorithm. By leveraging MQL4’s capabilities on MT4, you can create bots that implement sophisticated trading strategies, including trend-following systems or mean-reversion models. Whether you are new to algorithmic trading or experienced in coding, mastering MQL4 and MQL5 opens up numerous possibilities for automating your trading process and optimizing performance in dynamic market conditions.
Overview of Trading Bot
A trading bot is an automated software program designed to execute trading strategies on financial markets without human intervention. These bots can monitor market conditions, analyze data, and place buy or sell orders based on predefined rules. The primary goal of a trading bot is to execute trades more efficiently, reduce human error, and take advantage of market opportunities that may arise 24/7. They can be programmed to follow a wide variety of strategies, such as trend-following, mean-reversion, or scalping, depending on the trader’s goals. Trading bots are commonly used in forex, stock, and cryptocurrency markets, where they can process large amounts of market data quickly and make split-second decisions. They operate through APIs (Application Programming Interfaces) that allow them to connect directly to trading platforms like MetaTrader 4 or 5, executing trades based on real-time market conditions.
Basics of MQL4 and MQL5
MQL4 (MetaQuotes Language 4) and MQL5 (MetaQuotes Language 5) are programming languages designed specifically for creating trading robots, custom indicators, scripts, and automated strategies for use on the MetaTrader trading platforms (MT4 for MQL4 and MT5 for MQL5). Both languages are similar in structure but differ in their capabilities and use cases, with MQL5 offering more advanced features and a broader range of functions compared to MQL4.
MQL4 Basics:
MQL4 is primarily used to develop expert advisors (EAs), which are automated trading bots for MetaTrader 4. The language follows a C-like syntax and provides access to a variety of built-in functions for order management, market analysis, and indicators. MQL4 supports essential programming concepts such as variables, functions, loops, and conditions, making it relatively easy for beginners to learn. The key events in MQL4 are OnTick, which runs when a new tick (price change) occurs, and OnInit, which initializes the expert advisor when it’s loaded on a chart.
MQL5 Basics:
MQL5, the successor to MQL4, was introduced with MetaTrader 5 and offers enhanced capabilities, including a more robust object-oriented programming (OOP) approach. MQL5 allows for more complex trading strategies, and it supports more types of orders and advanced features like multi-threading and backtesting with greater accuracy. Like MQL4, MQL5 uses functions like OnTick and OnInit, but it also introduces additional events such as OnTimer and OnTrade, providing more flexibility for managing trades and operations. The object-oriented approach in MQL5 makes it easier to organize complex code, and it allows for greater reuse of code through classes and objects. While MQL5 is more powerful, it can be more challenging for beginners due to its advanced features.
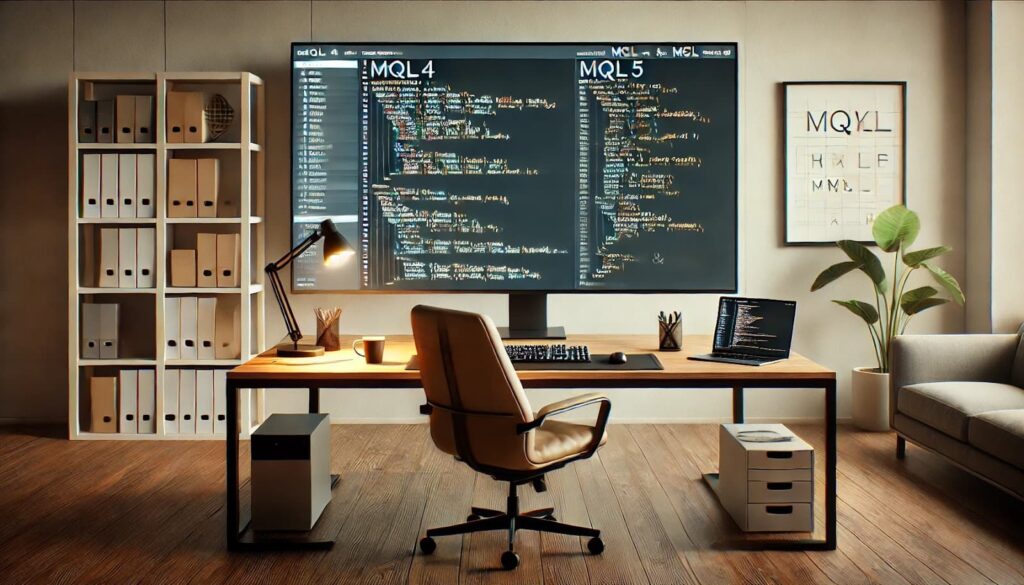
Best Coding Practices in MQL4/MQL5
When coding in MQL4 and MQL5, adhering to best coding practices is essential for creating efficient, reliable, and maintainable trading bots and indicators. Below are some of the best coding practices to follow when working with these languages:
1. Clear and Descriptive Naming Conventions
- Variables: Use meaningful variable names that describe their purpose, e.g., lotSize, stopLoss, takeProfit, movingAveragePeriod, rather than vague names like var1 or temp.
- Functions: Function names should indicate what they do, e.g., CalculateMovingAverage or PlaceBuyOrder.
- Constants: Use uppercase letters with underscores for constants, e.g., MAX_ORDERS.
2. Commenting and Documentation
Code Comments: Always document your code with comments explaining the purpose of functions, complex logic, and variables. This will help you and others understand the code later. For example:
// This function calculates the simple moving average
double CalculateMovingAverage(int period) {
// Code logic here
}
- Function Documentation: At the beginning of each function, provide a description of its purpose, parameters, and return values. This is especially important for larger projects.
3. Modular and Reusable Code
- Break Down Code into Functions: Use functions to modularize your code. This makes it more readable and easier to debug. For example, create separate functions for calculating indicators, placing orders, and handling errors.
- Avoid Repetition: If you find yourself writing the same code multiple times, encapsulate it in a function. This makes it easier to update the code if needed.
4. Error Handling and Logging
Check for Errors: Always check the return values of functions like OrderSend, OrderClose, and OrderModify. If an error occurs, log it using the Print or Alert functions to help with debugging.
int ticket = OrderSend(symbol, OP_BUY, lotSize, Ask, slippage, stopLoss, takeProfit, “Buy Order”);
if(ticket < 0) {
Print(“Error in OrderSend: “, GetLastError());
}
- Use Logging: Use the Print function or custom logging functions to record important information and errors during runtime, which is invaluable for debugging and improving your code.
5. Optimization and Efficiency
- Avoid Unnecessary Loops: Avoid unnecessary calculations or loops inside the OnTick or OnInit functions. For example, if the bot only needs to check a condition once every minute, avoid checking it on every tick.
- Minimize Resource Usage: Since trading bots often run continuously, optimize the code to use minimal resources. For example, use Sleep() function where applicable to avoid excessive CPU usage.
6. Risk Management and Safety
- Implement Stop-Loss and Take-Profit: Always implement proper risk management rules in your trading algorithms. Ensure that your trading bot places stop-loss and take-profit orders at appropriate levels to manage risk effectively.
- Avoid Hard-Coded Values: Instead of hard-coding critical parameters like stop-loss levels, take-profit, and lot sizes, make them configurable or parameterized so that they can be easily adjusted.
How to Code a Trading Bot with MQL4 and MQL5
Creating a trading bot using MQL4 and MQL5 involves several key steps, from setting up your development environment to coding, testing, and deploying the bot. Below is a step-by-step guide on how to code a trading bot with MQL4 (for MetaTrader 4) and MQL5 (for MetaTrader 5).
1. Set Up Your Development Environment
- Install MetaTrader 4/5: To start coding, you need to have MetaTrader 4 (MT4) or MetaTrader 5 (MT5) installed on your computer. You can download these platforms from the official MetaTrader website or a broker’s site.
- Launch MetaEditor: MetaEditor is the integrated development environment (IDE) for writing and testing MQL4/MQL5 code. You can open it directly from MetaTrader by going to “Tools” and selecting “MetaEditor”.
- Create a New Expert Advisor (EA): Once in MetaEditor, create a new project by selecting “File” > “New” > “Expert Advisor (template)” for MQL4 or MQL5. This template provides the basic structure for a trading bot.
2. Understand the Basic Structure
A trading bot in MQL4 or MQL5 typically consists of several main parts:
- OnInit(): Initializes the bot when it’s attached to a chart. Used for setting up initial parameters.
- OnTick(): This is the heart of your trading bot. It runs every time the market receives a new price tick (or update). In this function, you’ll check for market conditions and make trading decisions.
- OnDeinit(): Cleans up when the bot is removed from the chart.
Here’s an example of the basic structure of an EA:
// MQL4/MQL5 basic template
int OnInit() {
// Initialization code
Print(“Bot initialized”);
return INIT_SUCCEEDED;
}
void OnTick() {
// Main logic for trading
if (CheckBuyConditions()) {
PlaceBuyOrder();
}
else if (CheckSellConditions()) {
PlaceSellOrder();
}
}
void OnDeinit(const int reason) {
// Cleanup code
Print(“Bot removed”);
}
bool CheckBuyConditions() {
// Define conditions for a buy order
return (SomeIndicatorCondition() > 50);
}
bool CheckSellConditions() {
// Define conditions for a sell order
return (SomeIndicatorCondition() < 50);
}
void PlaceBuyOrder() {
// Place a buy order (simplified example)
double lotSize = 0.1;
double stopLoss = 50;
double takeProfit = 100;
int ticket = OrderSend(Symbol(), OP_BUY, lotSize, Ask, 2, stopLoss, takeProfit, “Buy Order”, 0, 0, Green);
if (ticket < 0) {
Print(“Error placing buy order: “, GetLastError());
}
}
void PlaceSellOrder() {
// Place a sell order (simplified example)
double lotSize = 0.1;
double stopLoss = 50;
double takeProfit = 100;
int ticket = OrderSend(Symbol(), OP_SELL, lotSize, Bid, 2, stopLoss, takeProfit, “Sell Order”, 0, 0, Red);
if (ticket < 0) {
Print(“Error placing sell order: “, GetLastError());
}
}
3. Defining Trading Conditions
- Indicators: Your bot needs to make decisions based on technical analysis, and MQL4/MQL5 provides a wide range of built-in indicators (e.g., Moving Average, RSI, MACD). You can access these indicators using functions like iMA (for Moving Average) or iRSI (for RSI).
- Trade Conditions: The OnTick() function will evaluate your conditions, such as whether the price crosses a moving average, or if the RSI is above 70 (overbought) or below 30 (oversold). Once a condition is met, the bot will place an order.
Example of a simple condition using a Moving Average:
double maCurrent = iMA(Symbol(), 0, 14, 0, MODE_SMA, PRICE_CLOSE, 0); // 14-period moving average
double maPrevious = iMA(Symbol(), 0, 14, 0, MODE_SMA, PRICE_CLOSE, 1);
if (maCurrent > maPrevious) {
// Place buy order
} else if (maCurrent < maPrevious) {
// Place sell order
}
4. Order Management
- Placing Orders: You can place buy or sell orders using functions like OrderSend() in MQL4 or OrderSendAsync() in MQL5.
- Stop Loss and Take Profit: Always implement stop-loss and take-profit levels to manage risk. For instance, OrderSend() allows you to specify stop-loss and take-profit levels in points.
5. Risk Management
- Lot Size: Ensure that you are calculating lot sizes correctly based on your available balance and desired risk parameters.
- Trailing Stop: Implement a trailing stop mechanism to lock in profits as the market moves in your favor.
Example of a simple risk management system:
double riskAmount = 100; // Define risk amount in account currency
double accountBalance = AccountBalance();
double lotSize = riskAmount / (stopLoss * MarketInfo(Symbol(), MODE_POINT) * AccountLeverage());
6. Backtesting Your Trading Bot
- Strategy Tester: MetaTrader’s built-in Strategy Tester allows you to backtest your trading bot using historical market data. This is crucial to evaluate how the bot performs over time before deploying it in live conditions.
- Optimization: Use the Strategy Tester’s optimization feature to find the best parameters for your trading strategy, such as the optimal moving average period or stop-loss levels.
7. Deployment
- Deploy on a Demo Account: Always test your trading bot on a demo account first to ensure that it behaves as expected under live market conditions.
- Monitoring and Adjustments: Once deployed on a live account, continuously monitor the bot’s performance. You may need to make adjustments based on market conditions or performance results.
8. Advanced Features
- Multiple Timeframes: You can code your bot to check conditions on different timeframes to make more informed trading decisions.
- Multiple Indicators: Combine different technical indicators to improve the accuracy of your trading signals.
- Advanced Order Types: In MQL5, you can use more advanced order types such as market, limit, stop, and trailing stop orders.
9. Optimizing Performance
- Efficient Code: Make sure your code runs efficiently. Avoid excessive calculations in the OnTick() function and optimize loops where possible to reduce CPU usage.
- Error Handling: Ensure you have proper error handling and logging in place to troubleshoot issues if they arise.
Conclusion
Coding a trading bot using MQL4 and MQL5 is a highly effective way to automate trading strategies and improve efficiency in the financial markets. By leveraging the power of MetaTrader platforms and their respective programming languages, traders can create bots that can analyze market conditions, execute trades, and manage risk autonomously. Understanding the core structure of MQL4 and MQL5, along with proper implementation of indicators, order management, and risk controls, is key to developing a successful trading bot.